Welcome to the CrowdSec Documentation
CrowdSec provides open source solution for detecting and blocking malicious IPs, safeguarding both infrastructure and application security.
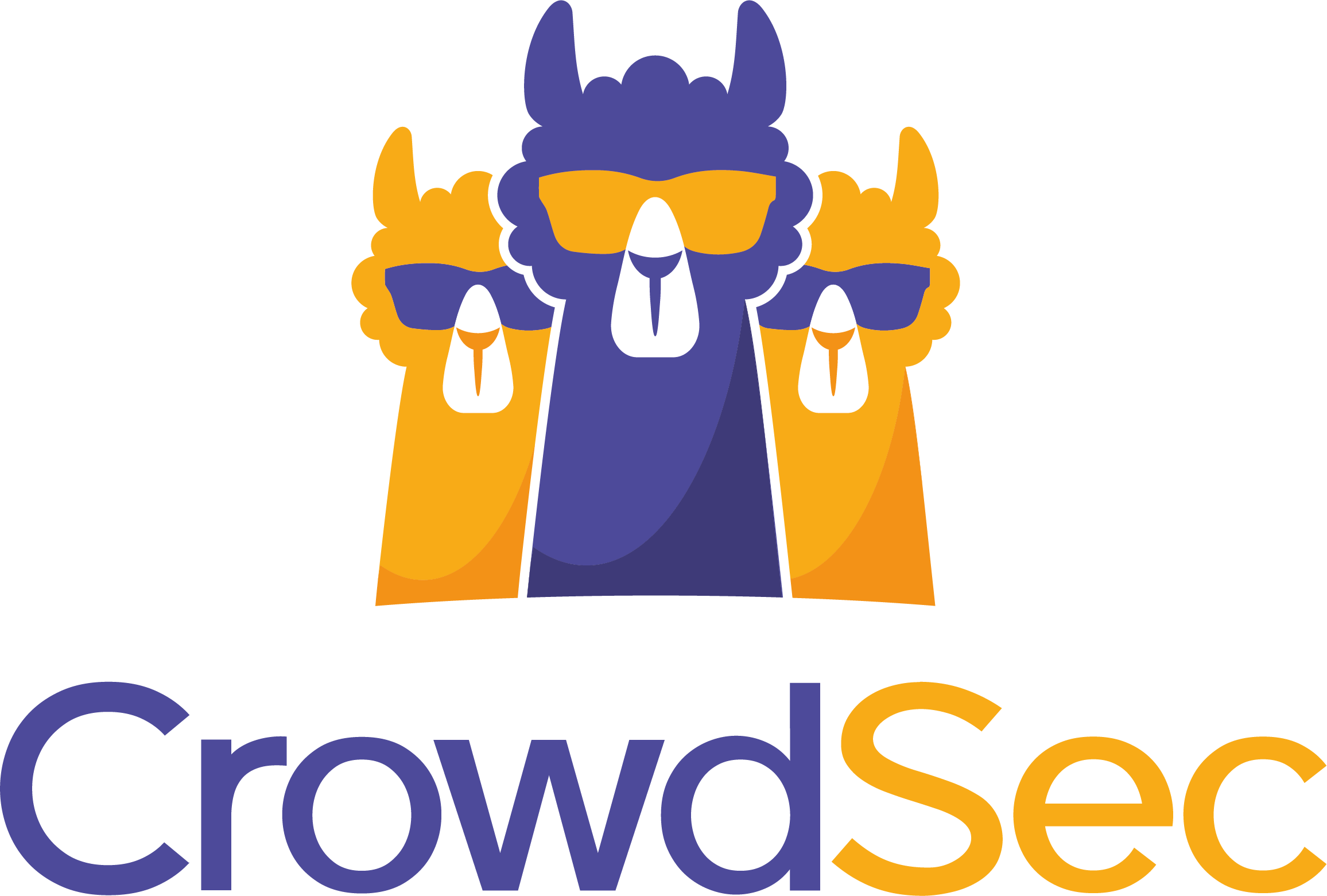
Select your environment
We can secure your stack. Just select your platform and get started.
*Logos and trademarks, such as the logos above, are the property of their respective owners and are used here for identification purposes only.
Get to know us!
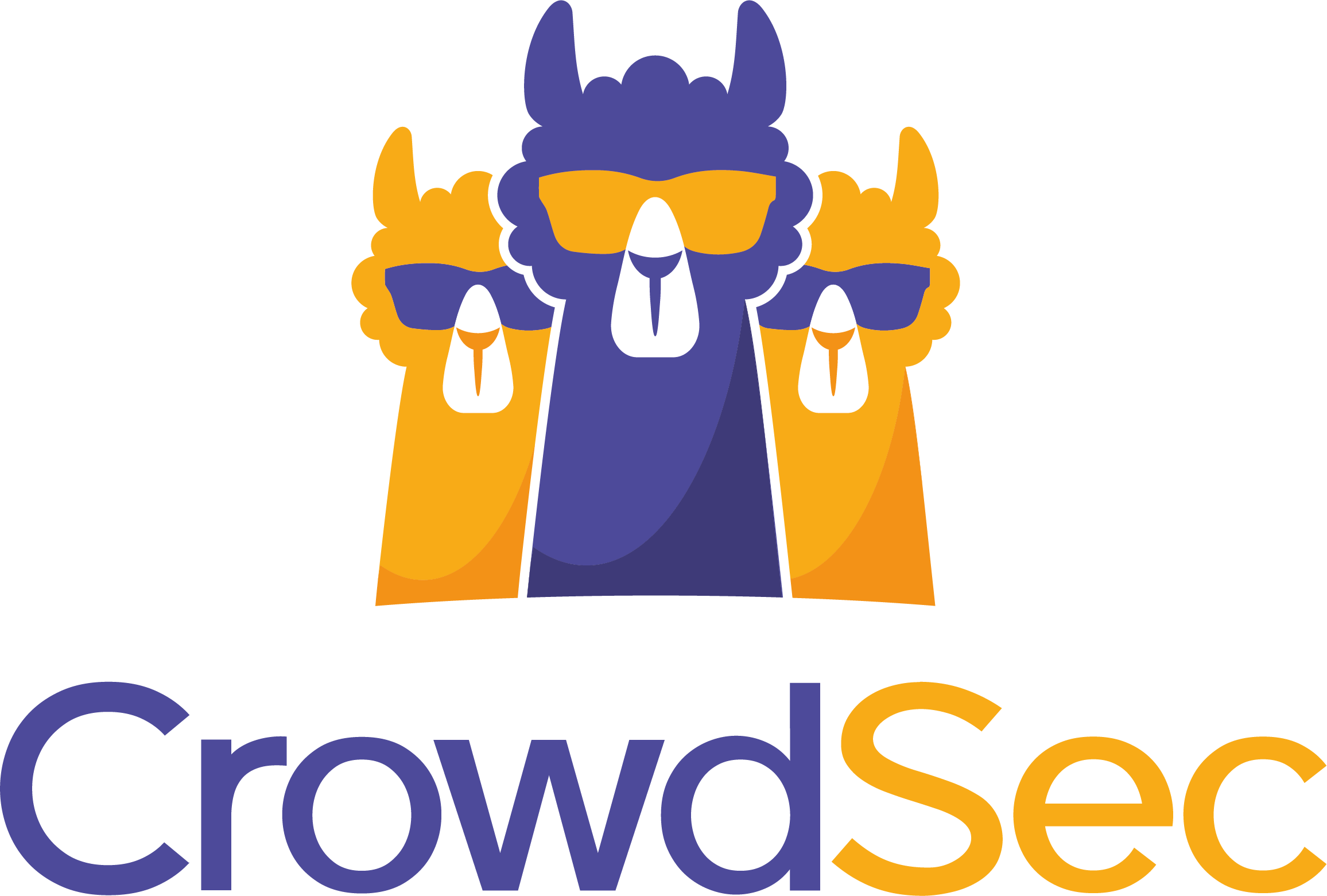
What is CrowdSec?
Data curated solution with a bunch of millions IPs detected by our large community.
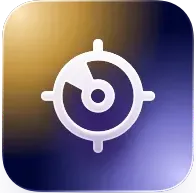
Security Engines
Secure yourself.
🖥️
CrowdSec Console
Manage and monitor your security.
🧑🏻💻
CrowdSec CLI
Use our command line interface.
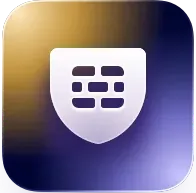
CrowdSec WAF
Protect your web applications.
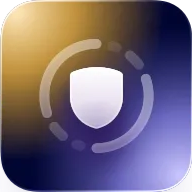
Blocklists
Block thousands of IPs.
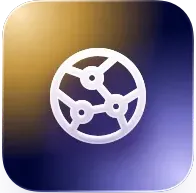
APIs
Integrate with your tools.